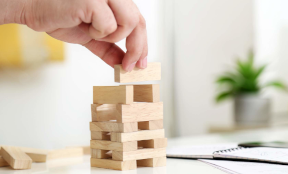
Configuring and setting up the VSB and VIP is known as platform development.
After this section you will be able to:
The kernel shell can use two modes: the C interpreter and the command interpreter. To switch between the two modes, you need to type the name of the interpreter into the shell. C for the C interpreter and cmd for the command interpreter.
-> cmd
[vxWorks *]# C
->
You can also type an interpreter's name before a command to execute a single command using that interpreter.
-> cmd
[vxWorks *]# C x=42
New Symbol “x” added to kernel symbol table.
value = 42 = 0x2a = '*'
[vxWorks *]# C
->
Type C (upper case) to start the C interpreter.
The command syntax is generally the same as in the C language, as show in this example.
The C interpreter has some syntax differences.
You can omit function call parentheses if the entire expression is the call. For example:
-> func &mac, 27
not:
-> 2 * func &mac, 27
The shell does not recognize symbolic macros.
Do not enter symbolic macros.
-> sem1 = semMCreate(SEM_Q_PRIORITY)
Enter
-> sem1 = semMCreate(0x1)
- There is no support for C control structures (for, while, if, and so on) and the shell does not recognize data structures.
Type cmd to change to the command interpreter.
This is a list of the most common commands for working with RTPs:
The command interpreter supports common shell control characters:
There are a few commands worth always having ready, these range from debugging, memory management, to analytics.
The dynamic printf command or dprintf is particularly useful if it is unclear where to set breakpoints, as well as to find race conditions that are hidden when the application is stopped.
Displaying system information is a valuable tool when working a project, here is a list the most common commands:
devs() | Lists all devices known on the target system. |
lkup() | Takes a regular expression as an argument and will list symbols from the symbol tables. |
lkAddr() | Takes an address as a parameter and lists the symbols whose values are near the specified address. |
printErrno() | Describes the most recent error status value. |
Show functions display useful information about the target system. Invoke them from the C interpreter of the kernel shell. They must include the associated component in the VIP.